Why should Eloquent have all the fun?
The PR I just sent to @laravelphp adds a new helper function `without_recursion()`
This lets you prevent infinite recursion anywhere in your application... Also it opens some other interesting opportunities...
Check it out here: github.com/laravel/framework/pull/52865
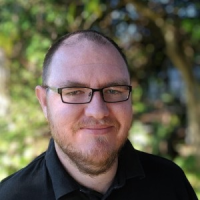
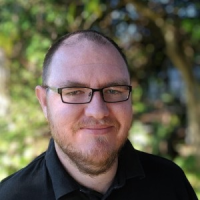
Samuel Levy
@samlev
Software architect and consultant, working on PHP/Laravel/Livewire
• • 20 Posts • 381 Views
Welp, I guess that pinkary needs a process for dealing with spam now :(
Eloquent inverse relations (a.k.a. `chaperone()`) and fixes for serializing circular references became available today with v11.22.0!
I was honestly surprised that these PRs got merged, then shocked to see Taylor showing them off in his keynote at #Laracon, but I'm happy that they're available now!
Time to update a bunch of my projects!
Opinions wanted: Which of these talk titles is most appealing to you?
1️⃣ Turning NoSQL into YeSQL
2️⃣ What's in a database? (Another database!)
3️⃣ YeSQL: But is it webscale?
4️⃣ Say YeSQL to multi-tenancy!
5️⃣ YeSQL: The unwanted answer to NoSQL
Working on my little TALL stack UI/component library... If you were installing it, would you prefer components to be namespaced and/or grouped?
1. <x-input />
2. <x-form.input />
3. <x-tallboy::input />
4. <x-tallboy::form.input />
Additional question: would you prefer to directly reference livewire components or have blade components that wrap them for consistency in your templates?
1. <livewire:tallboy.uploader />
2. <x-tallboy::uploader />